
- 2Configuration
VTK is cross-platform and runs on Linux, Windows, Mac and Unix platforms. Vtk ā VTK toolkit. Method:-iām assuming you already have JDK and a Java development environment Eclipse installed. In addition you will need to download the VTK source and build it. This requires a working C compiler. VTK wrapping on Windows involves the following steps (the process is similar on Linux or Mac): Download and install CMake 2.8 or 3.0 (has been tested with both) Download VTK. Check 'VTKWRAPJAVA' and set the correct paths to your Java SDK installation (if your building for 64bit these need to be 64bit). Click configure again. Using Java with VTK on Mac OS X 10.3 and higher is basically broken. This project aims to correct these problems and provide a version of VTK on the Mac where Java (and C) can be used. This code is based on the latest VTK 4.2 stable release. Hello, Java 11 has apparently some bugs (with vtk in particular), you recommend using java 1.8, but by default user would download the first version on the webp. VTK 4.2.2 / JAVA 1.4!! THE INSTRUCTIONS TO COMPILE ARE NOT YET AVAILABLE - Based on VTK 4.2.2 / Java 1.4 An old software wrote several years ago (1999 - 2002). Volumetric Rendering - Virtual endoscopy - Segmentation (extract/isolate parts from volum) - Pattern Search (intestinal polyps search).
Introduction
Some other documentation can be found here:- http://www.particleincell.com/2011/vtk-java-visualization
Configuration
You basically just need to turn VTK_WRAP_JAVA on in CMake and build.
Bartlomiej Wilkowski has created a nice tutorial of configuring Java wrapping with VTK.
Windows
To run a sample application provided in VTK against your VTK build directory (with an installed VTK remove 'Debug'):

Mac
To run a sample application provided in VTK against your VTK build directory (with an installed VTK replace 'bin' with 'lib'):
Linux
To run a sample application provided in VTK against your VTK build directory (with an installed VTK replace 'bin' with 'lib'):
Sample Code (from VTK/Wrapping/Java/vtk/sample/SimpleVTK.java)
Some key points from this code to note:
- vtkNativeLibrary.LoadAllNativeLibraries() is required to load the dynamic libraries from VTK's C++ core. This call must happen before any VTK code executes, which is why it is put in a static block in our application class.
- vtkNativeLibrary.DisableOutputWindow(null) simply hides any debugging information that may otherwise pop up in case of VTK error or warning. You can also provide a file path so any error will be written to disk.
- SwingUtilities.invokeLater(...) is called because technically all GUI code, including setting up and using a VTK render window, should happen in the Swing event thread.
Threading Sample Code (from VTK/Wrapping/Java/vtk/sample/Demo.java)
In this demo, we want to illustrate the correct way to perform VTK tasks on separate threads in Java. The first thing to note is that VTK is inherently NOT thread-safe, which immediately rules out several possible use cases. Calling methods on the same VTK objects across threads, even if they seem to be read-only, should be avoided. The safest approach is to 'hand off' objects from one thread to another, so one thread is completely done with an object before another thread begins manipulating it. Reclaiming memory for VTK objects is particularly tricky to perform across threads, as deleting a single VTK object may potentially cause the entirety of VTK objects to be modified. While we expose the Delete() method to explicitly delete VTK objects, if you are using VTK objects in multiple threads this is discouraged unless you are aware of its potential issues. VTK provides a special garbage collector for VTK objects in the Java layer that may be run manually or automatically at intervals if memory reclaiming is needed.
For this example, we will have a checkbox for turning on and off the VTK garbage collection while an application is running. The application creates new actors using a separate processing thread, which are then added dynamically to the VTK renderer. This enables data to be loaded and processed without causing lags in the frame rate of the interactive 3D view.
We need to implement a worker that is capable of producing actors. In the sample code we producesphere actors with shrunk polygons in order to have something interesting that takes a bit of time to create. These will execute on separate threads to keep the rendering interactive.
A separate worker's job is to add actors to the renderer when ready.
In our initialization code, we need to set up several things. First, two checkboxes toggle VTK's garbage collection and the debug mode. Since VTK is a C++ library, it has its own mechanism for ensuring that unused objects are deleted from memory. Many threading issues can be avoided by simply turning off VTK garbage collection.
We need to set up our completion service.
Next a setupWorkers() method starts a thread which invokes the code to add actors to the renderer whenever our executor completion service has a new actor available. Note that the code adding the actors to the renderer must be done on the event thread using SwingUtilities.invokeAndWait(), since that is where the renderer object was created and lives.
To load the completion service with jobs to run on a pool of threads in the background, we submit a collection of PipelineBuilder objects to be executed at a later time.
Vtk Python Example
We'll also create a timer which every second renders the scene and takes out a sphere actor. This code also manually run the garbage collector using vtkObject.JAVA_OBJECT_MANAGER.gc() if the runGC checkbox is selected. Note that timers are scheduled on the Swing event thread, which is why we are allowed to manipulate the renderer and its actors here. We call the garbage collector manually in order to update the UI with garbage collector information.
Instead of manually running the garbage collector, we can set up automatic garbage collection using a global scheduler. Turn on automatic garbage collection with the following statement. It is important to note that by default it is off, so be sure to include this line to reclaim memory from the VTK layer.
To set up the interval at which collection runs, use SetScheduleTime. In this case it will run garbage collection every second. The automatic garbage collector runs in the event thread by default.
Another option will collect statistics on the garbage collector, that can be retrieved by listKeptReferenceToString() and listRemovedReferenceToString() on the collector's information object returned from the gc() method.
Java Wrapper Refactoring (Oct 8, 2007)
There were a few problems with the old Java wrappers. One was that, asyou said, objects were being deleted before they were supposed to. Wehacked in a fix at one point about a year ago which basically made allVTK objects accessed from Java stay around forever, but this was notacceptable either.
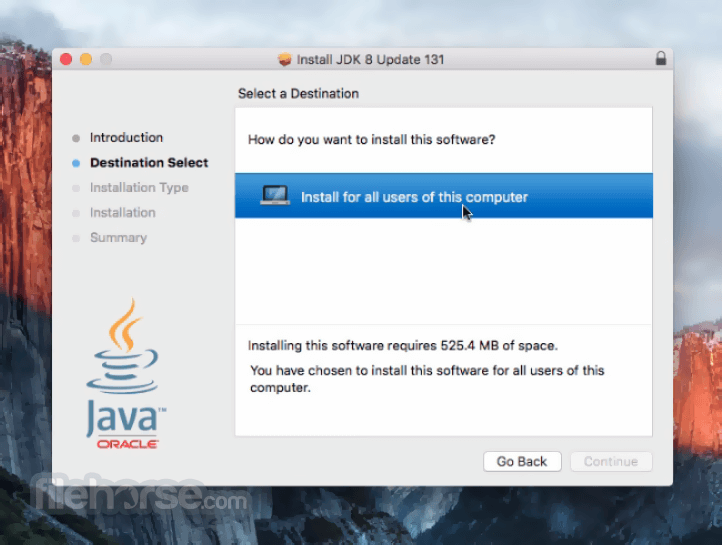
Ref:
The other major concern was that the map from Java objects to VTKobjects was in the C++ JNI layer, and while we tried to keep this mapsynchronized with a mutex, race conditions could still occur becauseother Java threads could advance while the JNI layer was being called(a thread could access a C++ object just as it is beinggarbage-collected and deleted). There does not seem to be a way toatomically call a JNI method, or ensure the collector doesn't runwhile a method is called. This second issue forced us to rethink howthe map is done, and the solution was to keep the map in Java insteadof C++. But we didn't want this Java map to prohibit objects frombeing garbage collected. Fortunately, Java has a WeakReference classfor just this type of purpose. When accessed, the reference willeither be valid or null depending on whether it has beengarbage-collected.
Thus, the wrapper code can lookup objects in this map when returningobjects from methods, and if it is not there, or null, it creates anew Java object representing that C++ object.
Vtk Library
A final issue was that we wanted a way to guarantee all C++destructors are called before the program exits. The natural place todecrement the reference count of the C++ object is in finalize(),which works when things are garbage-collected, but Java does notguarantee that finalize will ever be called. So the methodvtkGlobalJavaHash.DeleteAll() will plow through the remaining VTKobjects and call Delete on them.
Wrapping VTK for Java support is relatively easy and straight forward compared to ITK wrapping, however, it is also less important as ImageJ already brings a lot of rendering capability to the table. Nevertheless it may be useful for mesh smoothing, coarsening, or similar applications.
VTK wrapping on Windows involves the following steps (the process is similar on Linux or Mac):
Vtk 8.2 Downloads
- Download and install CMake 2.8 or 3.0 (has been tested with both)
- Download VTK (we have tested 6.1)
- Extract the source code to .../VTK-6.1/src/ and configure the project using CMake. We configured the project for Visual Studio 2010 Professional, 64bit, and Release mode. Make sure to uncheck 'Build examples' and 'Build testing' for faster compilation. Check 'Build shared libraries'. Click configure.
- Check 'VTK_WRAP_JAVA' and set the correct paths to your Java SDK installation (if your building for 64bit these need to be 64bit). Click configure again, and then generate.
- Open the VS solution generated by CMake, right click the ALL_BUILD project, and click: build. The project should now compile. If everything was setup correctly, there should be no errors.
- Include the VTK binary directory in your PATH environment variable: '.../VTK-6.1/build/release/bin'.
- Include '.../VTK-6.1/build/release/bin/vtk.jar' into your Java project and you should be good to go.
Vtk Download Mac Java Tutorial
Demo code and a more detailed tutorial can be found here.
